238 除自身以外数组的乘积
本文最后更新于:2022年4月9日 中午
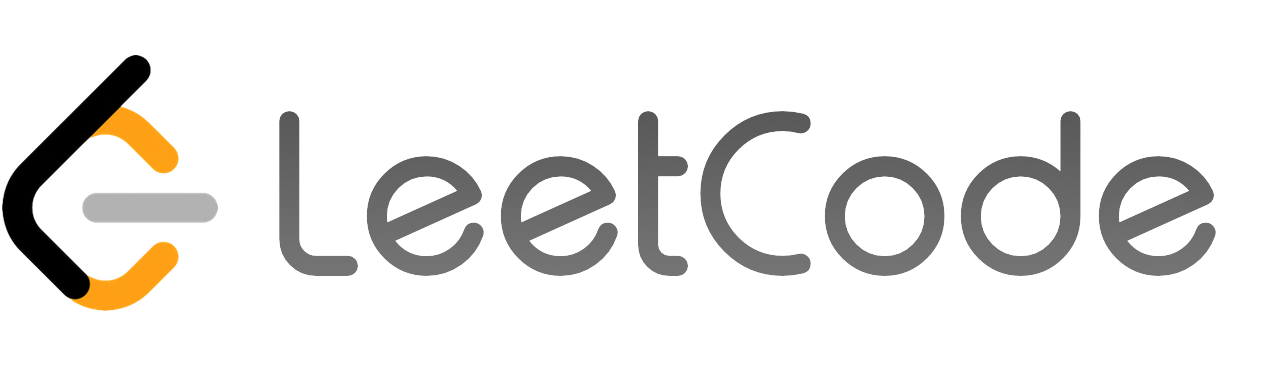
给你一个长度为 n 的整数数组 nums
,其中 n > 1,返回输出数组 output
,其中 output[i]
等于 nums
中除 nums[i]
之外其余各元素的乘积。
示例:
1 |
|
提示:题目数据保证数组之中任意元素的全部前缀元素和后缀(甚至是整个数组)的乘积都在 32 位整数范围内。
说明: 请不要使用除法,且在 O(n) 时间复杂度内完成此题。
进阶:
你可以在常数空间复杂度内完成这个题目吗?( 出于对空间复杂度分析的目的,输出数组不被视为额外空间。)
Solution
- 建立左右乘积列表
- 对于给定索引 i ,使用它左边所有数字的乘积乘以右边所有数字的乘积。
- 时间复杂度:O(N),其中 N 指的是数组
nums
的大小。 - 空间复杂度:O(N),其中 N 指的是数组
nums
的大小。
1 |
|
官方题解思路:
- 先把
answer
作为L
数组。 - 用一个遍历来跟踪右边元素的乘积。并更新数组
answer[i]=answer[i]*R
。然后 R 更新为R=R*nums[i]
,其中变量 R 表示的就是索引右侧数字的乘积。
1 |
|
本博客所有文章除特别声明外,均采用 CC BY-SA 4.0 协议 ,转载请注明出处!